Written by Koen Vervloesem, technology writer and author of the book Develop your own Bluetooth Low Energy Applications for Raspberry Pi, ESP32 and nRF52 with Python, Arduino and Zephyr.
This article originally ran on Koen Vervloesem’s blog, where you can find more content like this.
When I started learning how to develop Bluetooth Low Energy (BLE) applications with Zephyr RTOS, I was immediately impressed by the excellent documentation and the wealth of sample code of this open source real-time operating system. But what really got me understand the way of working with BLE on Zephyr was just going through each line of code in a sample and find out the corresponding definition in Zephyr’s header files, which are surprisingly readable.
As an exercise in this approach, I want to go through the iBeacon sample application with you. You’ll find that this deceptively simple application already hides a lot of details that are important to know before starting with more complex Zephyr code.
Here’s the sample code to broadcast an iBeacon advertisement:
/*
* Copyright (c) 2018 Henrik Brix Andersen <henrik@brixandersen.dk>
*
* SPDX-License-Identifier: Apache-2.0
*/
#include <zephyr/types.h>
#include <stddef.h>
#include <zephyr/sys/printk.h>
#include <zephyr/sys/util.h>
#include <zephyr/bluetooth/bluetooth.h>
#include <zephyr/bluetooth/hci.h>
#ifndef IBEACON_RSSI
#define IBEACON_RSSI 0xc8
#endif
/*
* Set iBeacon demo advertisement data. These values are for
* demonstration only and must be changed for production environments!
*
* UUID: 18ee1516-016b-4bec-ad96-bcb96d166e97
* Major: 0
* Minor: 0
* RSSI: -56 dBm
*/
static const struct bt_data ad[] = {
BT_DATA_BYTES(BT_DATA_FLAGS, BT_LE_AD_NO_BREDR),
BT_DATA_BYTES(BT_DATA_MANUFACTURER_DATA,
0x4c, 0x00, /* Apple */
0x02, 0x15, /* iBeacon */
0x18, 0xee, 0x15, 0x16, /* UUID[15..12] */
0x01, 0x6b, /* UUID[11..10] */
0x4b, 0xec, /* UUID[9..8] */
0xad, 0x96, /* UUID[7..6] */
0xbc, 0xb9, 0x6d, 0x16, 0x6e, 0x97, /* UUID[5..0] */
0x00, 0x00, /* Major */
0x00, 0x00, /* Minor */
IBEACON_RSSI) /* Calibrated RSSI @ 1m */
};
static void bt_ready(int err)
{
if (err) {
printk("Bluetooth init failed (err %d)\n", err);
return;
}
printk("Bluetooth initialized\n");
/* Start advertising */
err = bt_le_adv_start(BT_LE_ADV_NCONN, ad, ARRAY_SIZE(ad),
NULL, 0);
if (err) {
printk("Advertising failed to start (err %d)\n", err);
return;
}
printk("iBeacon started\n");
}
void main(void)
{
int err;
printk("Starting iBeacon Demo\n");
/* Initialize the Bluetooth Subsystem */
err = bt_enable(bt_ready);
if (err) {
printk("Bluetooth init failed (err %d)\n", err);
}
}
First, this includes a couple of header files. Then the code defines the IBEACON_RSSI constant, which sets the measured power in the iBeacon advertisement. The value 0xc8 is equal to decimal value 200. As this field in the iBeacon specification’s advertising data structure is encoded as a signed integer, this is interpreted as 200 – 256 = -56 dBm.
Advertising data structures in Zephyr
Then, you see the definition of an array of struct bt_data elements. The bt_data struct describes an advertising data structure with a type, length, and a pointer to the data. Its definition (in zephyr/bluetooth/bluetooth.h) is:
* advertising data. Used to form arrays that are passed to the
* bt_le_adv_start() function.
*/
struct bt_data {
uint8_t type;
uint8_t data_len;
const uint8_t *data;
};
In the iBeacon program, the array consists of two elements. This is consistent with the iBeacon specification, which shows that an iBeacon advertising packet consists of two advertising structures: flags and manufacturer-specific data.
In principle, you could fill the array with bt_data structs you create yourself, but Zephyr defines some helper macros. Their definition (again, from zephyr/bluetooth/bluetooth.h) looks like this:
/** @brief Helper to declare elements of bt_data arrays
*
* This macro is mainly for creating an array of struct bt_data
* elements which is then passed to e.g. @ref bt_le_adv_start().
*
* @param _type Type of advertising data field
* @param _data Pointer to the data field payload
* @param _data_len Number of bytes behind the _data pointer
*/
#define BT_DATA(_type, _data, _data_len) \
{ \
.type = (_type), \
.data_len = (_data_len), \
.data = (const uint8_t *)(_data), \
}
/** @brief Helper to declare elements of bt_data arrays
*
* This macro is mainly for creating an array of struct bt_data
* elements which is then passed to e.g. @ref bt_le_adv_start().
*
* @param _type Type of advertising data field
* @param _bytes Variable number of single-byte parameters
*/
#define BT_DATA_BYTES(_type, _bytes...) \
BT_DATA(_type, ((uint8_t []) { _bytes }), \
sizeof((uint8_t []) { _bytes }))
So, with the BT_DATA macro, you construct a bt_data struct with the type, data, and data length you specify (using a pointer to the data). BT_DATA_BYTES is a macro that uses the BT_DATA macro and automatically fills it with the right length for data with a known size.
If you return to the iBeacon program, you see that it fills the array with two elements using the BT_DATA_BYTES macro.
The first element is of type BT_DATA_FLAGS and with data BT_LE_AD_NO_BREDR. You can find the definition of these constants in zephyr/bluetooth/gap.h (GAP stands for Generic Access Profile). For non-connectable advertising packets, the flags data structure is optional.
The second element is of type BT_DATA_MANUFACTURER_DATA. The data used to construct this element follows the format listed in the iBeacon specification: Apple’s two-byte company ID, the type (0x02), and length (0x15 or 21 bytes) for the iBeacon data type, and then the UUID, major, minor, and measured power.
All in all, the result of all these macros is an array with advertising data structures that looks like this, schematically:
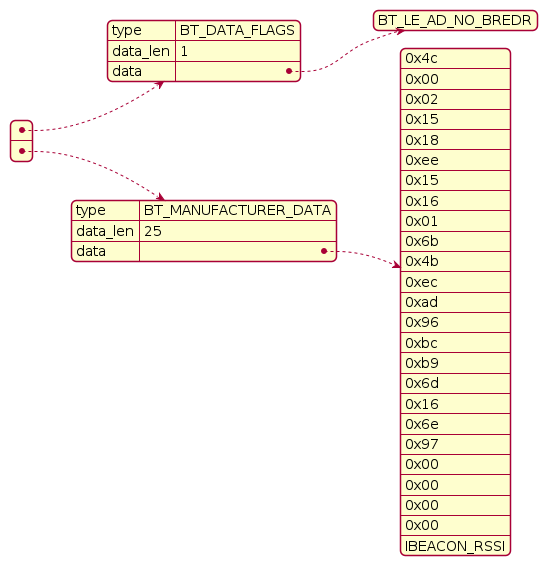
Enabling Bluetooth
Until now, the code has been all about setting up the right data structures to advertise. Let’s have a look at the main() function. This actually does just one thing: calling the bt_enable() function. You have to call this function before any other calls that use the board’s Bluetooth hardware. It initializes the Bluetooth subsystem and returns 0 on success and a negative error code otherwise.
The argument to the bt_enable() function is a callback function that’s called when initializing Bluetooth is completed. It’s in this callback function, bt_ready(), that the core of the program resides.
Advertising
The callback function is called with one argument — an error code that is 0 on success, or an error code consisting of a negative value otherwise. So, in bt_ready(), you first check whether Bluetooth initialization failed, and, if not, you start advertising.
The definition of bt_le_adv_start() (still in zephyr/bluetooth/bluetooth.h), the function used to start advertising, is:
/** @brief Start advertising
*
* Set advertisement data, scan response data, advertisement parameters
* and start advertising.
*
* When the advertisement parameter peer address has been set the advertising
* will be directed to the peer. In this case advertisement data and scan
* response data parameters are ignored. If the mode is high duty cycle
* the timeout will be @ref BT_GAP_ADV_HIGH_DUTY_CYCLE_MAX_TIMEOUT.
*
* @param param Advertising parameters.
* @param ad Data to be used in advertisement packets.
* @param ad_len Number of elements in ad
* @param sd Data to be used in scan response packets.
* @param sd_len Number of elements in sd
*
* @return Zero on success or (negative) error code otherwise.
* @return -ENOMEM No free connection objects available for connectable
* advertiser.
* @return -ECONNREFUSED When connectable advertising is requested and there
* is already maximum number of connections established
* in the controller.
* This error code is only guaranteed when using Zephyr
* controller, for other controllers code returned in
* this case may be -EIO.
*/
int bt_le_adv_start(const struct bt_le_adv_param *param,
const struct bt_data *ad, size_t ad_len,
const struct bt_data *sd, size_t sd_len);
So, to call this function, you need to supply advertising parameters, the advertising data structure’s array and its length, as well as the array of data structures for the scan response packet and its length.
The iBeacon example program doesn’t use any scan response data, so the last two arguments are NULL and 0. The array of advertising data structures is ad, which you constructed in the beginning of the code. With ARRAY_SIZE, a macro defined in zephyr/sys/util.h, you compute the number of elements in the array. When using a C compiler, this macro is defined as:
#define ARRAY_SIZE(array) (sizeof(array) / sizeof((array)[0]))
The first argument to the bt_le_adv_start() function specifies the advertising parameters. In this example, this argument is BT_LE_ADV_NCONN. If you look up its definition in zephyr/bluetooth/bluetooth.h, you’ll see that this defines non-connectable advertising with a private address:
/** Non-connectable advertising with private address */
#define BT_LE_ADV_NCONN BT_LE_ADV_PARAM(0, BT_GAP_ADV_FAST_INT_MIN_2, \
BT_GAP_ADV_FAST_INT_MAX_2, NULL)
The macro BT_LE_ADV_PARAM is another helper macro:
/**
* @brief Helper to declare advertising parameters inline
*
* @param _options Advertising Options
* @param _int_min Minimum advertising interval
* @param _int_max Maximum advertising interval
* @param _peer Peer address, set to NULL for undirected advertising or
* address of peer for directed advertising.
*/
#define BT_LE_ADV_PARAM(_options, _int_min, _int_max, _peer) \
((struct bt_le_adv_param[]) { \
BT_LE_ADV_PARAM_INIT(_options, _int_min, _int_max, _peer) \
This teaches you that BT_GAP_ADV_FAST_INT_MIN_2 and BT_GAP_ADV_FAST_INT_MAX_2 are the minimum and maximum advertising interval. You can find their definitions in zephyr/bluetooth/gap.h:
#define BT_GAP_ADV_FAST_INT_MIN_2 0x00a0 /* 100 ms */
#define BT_GAP_ADV_FAST_INT_MAX_2 0x00f0 /* 150 ms */
So, your iBeacon will use an advertising interval of between 100 ms and 150 ms.
BT_LE_ADV_PARAM uses another macro in the same header file, BT_LE_ADV_PARAM_INIT, and its definition is:
/**
* @brief Initialize advertising parameters
*
* @param _options Advertising Options
* @param _int_min Minimum advertising interval
* @param _int_max Maximum advertising interval
* @param _peer Peer address, set to NULL for undirected advertising or
* address of peer for directed advertising.
*/
#define BT_LE_ADV_PARAM_INIT(_options, _int_min, _int_max, _peer) \
{ \
.id = BT_ID_DEFAULT, \
.sid = 0, \
.secondary_max_skip = 0, \
.options = (_options), \
.interval_min = (_int_min), \
.interval_max = (_int_max), \
.peer = (_peer), \
}
All in all, this means that the code starts non-connectable advertising with its default Bluetooth ID, a private address, no special advertising options, and with an advertising interval of between 100 ms and 150 ms.
Summary
When reading source code of Zephyr applications, you can definitely feel discouraged by all these macros at first. However, after you’ve seen a couple of Zephyr programs, you start to see how they all fit together. The beauty of Zephyr is that its source code is completely open. If you don’t understand what a specific macro or function is doing, just look up its declaration in the header file or even in the corresponding C file where it’s implemented.
This approach has thought me a lot about developing Bluetooth Low Energy applications with Zephyr. I hope it will be a useful approach to you too.